Unlocking the potential of programming languages starts with understanding how they work.
In today’s rapidly evolving tech landscape, understanding the inner workings of programming languages is essential for both new and seasoned developers. Each language has its own architecture and execution model that shapes how code is processed, how it interacts with the system, and how developers utilize it to build applications. This blog explores the three major programming paradigms: compiled, bytecode, and interpreted languages, focusing on C++, Java, and Python, respectively.
The Basics of Programming Languages
At their core, programming languages provide a way for developers to communicate instructions to a computer. These instructions are ultimately executed by the hardware, which understands only machine code—binary instructions that the CPU can process. However, writing code in binary is impractical for humans, leading to the development of higher-level programming languages. Each language has its own syntax, semantics, and execution model, determining how code is translated into machine-readable instructions.
Compiled Languages: C++
C++ is a prime example of a compiled language. When you write code in C++, you are creating source code, which must be transformed into machine code before it can be executed. This transformation is achieved through a process called compilation.
Compilation Process
- Preprocessing: Before the compilation begins, the preprocessor takes care of directives (like
#include
for including libraries) and macros (defined using#define
). This step prepares the code for the next stage. - Compilation: The compiler converts the preprocessed code into assembly language, which is a human-readable representation of machine code. The output at this stage is usually an object file (.obj or .o) containing machine code but not yet fully linked.
- Linking: The linker takes one or more object files and combines them into a single executable file. It resolves references between different object files and libraries to create a complete program.
- Execution: The resulting executable file can be run directly by the operating system. Since it is in machine code, the CPU can execute the instructions without any further translation.
Advantages and Disadvantages
The main advantage of compiled languages like C++ is performance. Since the code is translated directly into machine code, execution tends to be faster compared to languages that require additional steps. However, this speed comes with a trade-off: C++ programs can take longer to compile, and any changes in the code require a recompilation of the affected modules.
Another disadvantage is platform dependence. Compiled code is specific to the architecture it was compiled for; thus, if you want to run a C++ program on a different architecture (e.g., moving from Windows to Linux), you need to recompile the code for that environment.
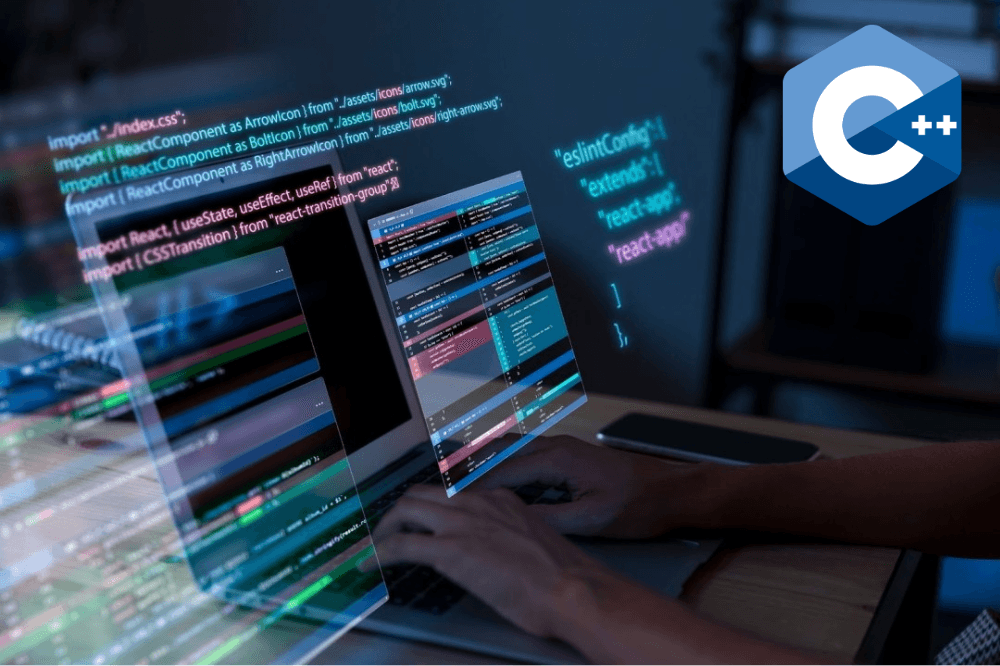
Bytecode Languages: Java
Java operates on a different paradigm, utilizing a combination of compilation and interpretation through a process that produces bytecode. This bytecode is designed to be executed on a Java Virtual Machine (JVM), making Java a platform-independent language.
Compilation and Execution Process
- Source Code: Like C++, Java begins with source code written in
.java
files. - Compilation: The Java compiler (javac) compiles the source code into bytecode, which is stored in
.class
files. This bytecode is not machine-specific; instead, it is a low-level representation that can be executed on any platform that has a JVM. - Execution via JVM: When you run a Java program, the JVM takes the bytecode and interprets it. The JVM is responsible for converting the bytecode into machine code. This can happen in two ways:
- Interpretation: The JVM reads and executes bytecode line by line. While this allows for flexibility and platform independence, it can be slower than executing machine code directly.
- Just-In-Time Compilation (JIT): Many modern JVMs include a JIT compiler that converts bytecode into native machine code at runtime. This allows for optimized execution and speeds up the performance of Java applications significantly, especially after the code has been executed multiple times.
Advantages and Disadvantages
The primary advantage of Java’s bytecode approach is platform independence. Developers can write Java code once and run it anywhere that a JVM is available, promoting portability. The JIT compilation further enhances performance by optimizing frequently executed paths in the code.
However, the need for a JVM can introduce overhead, making Java slower than fully compiled languages like C++. Additionally, while the JIT compiler improves performance, it can introduce unpredictability, as execution times may vary based on runtime conditions.
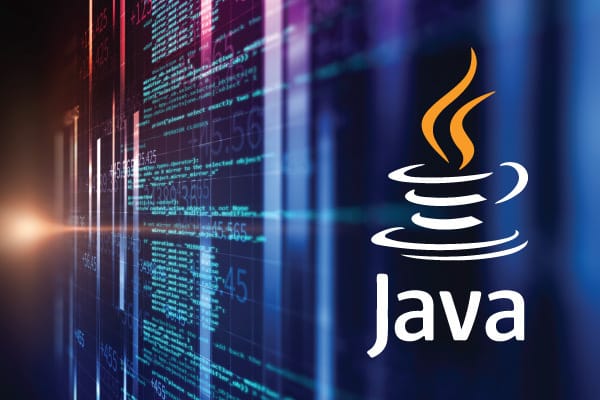
Interpreted Languages: Python
Python is widely recognized as an interpreted language. When you run a Python program, the code is executed by an interpreter at runtime without the need for prior compilation into machine code.
Execution Process
- Source Code: Python code is written in
.py
files, which contain human-readable instructions. - Interpretation: When a Python program is executed, the Python interpreter reads the source code and translates it into machine code on-the-fly. This process includes parsing the code, generating an abstract syntax tree (AST), and then executing the instructions.
- Bytecode Compilation: While Python is primarily an interpreted language, it does compile the source code into bytecode (stored in
.pyc
files) before execution. This bytecode is then executed by the Python virtual machine (PVM). However, this step is usually abstracted away from the user, making it feel like a purely interpreted language. [For a deeper understanding of how Python’s execution works, check out this detailed article on the Python Virtual Machine (PVM).]
Advantages and Disadvantages
The major advantage of Python’s interpreted nature is flexibility. Developers can quickly write and test code, making Python particularly appealing for rapid application development, scripting, and data analysis. The ability to run code directly without a compilation step allows for an interactive development experience, where developers can experiment and iterate swiftly.
However, this flexibility comes at a cost. Interpreted languages like Python often exhibit slower execution speeds compared to compiled languages. The line-by-line execution adds overhead, and while the bytecode compilation helps, it does not eliminate the performance gap entirely. Python’s dynamic typing can also lead to runtime errors that would be caught at compile-time in statically typed languages.
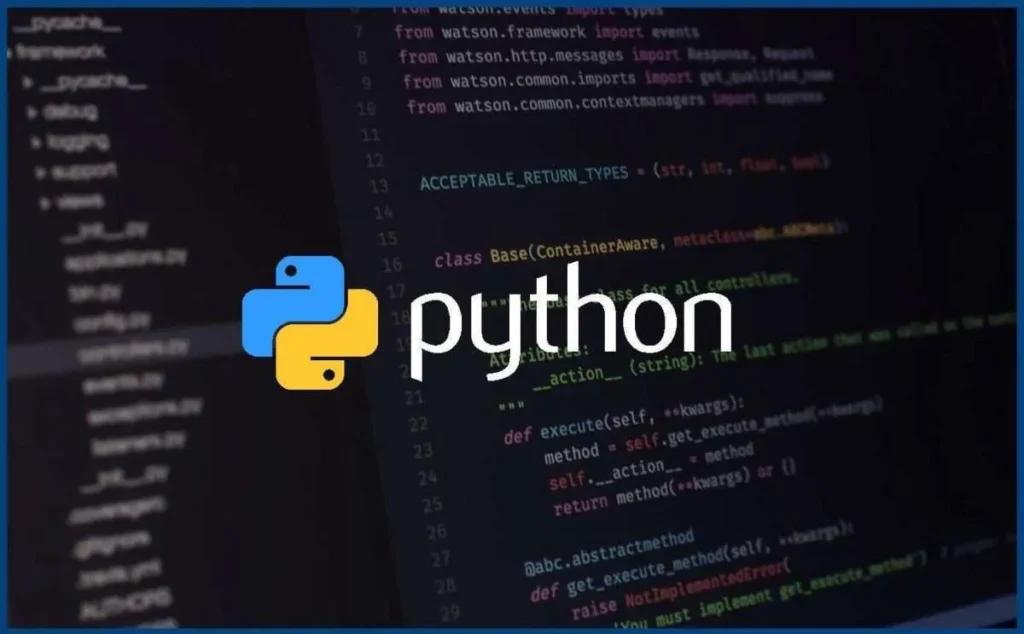
Summary: The Trade-offs of Different Execution Models
Understanding how C++, Java, and Python work reveals the trade-offs involved in choosing a programming language. Here’s a quick summary of the key differences:
Feature | C++ | Java | Python |
---|---|---|---|
Execution Model | Compiled | Bytecode (JVM) | Interpreted |
Performance | Generally fastest | Fast (especially with JIT) | Slower than compiled languages |
Portability | Platform-dependent | Platform-independent | Platform-independent |
Development Speed | Slower due to compilation | Moderate (compile step needed) | Fast (no compilation needed) |
Error Detection | Compile-time | Compile-time | Runtime |
Use Cases | Systems programming, games | Enterprise applications | Web development, data science |
In conclusion, the choice between C++, Java, and Python ultimately depends on the specific needs of a project. Developers should consider factors such as performance requirements, development speed, and the target platform when selecting a programming language. By understanding how each language works under the hood, developers can make informed decisions that align with their goals and project requirements.
Whether you’re building high-performance applications, enterprise solutions, or quick prototypes, the diverse capabilities of these languages provide the tools necessary to tackle a wide range of programming challenges.
MyceliumWeb specializes in software development and programming education, offering innovative solutions that focus on performance and scalability.
Ready to enhance your understanding of C++, Java, and Python? Contact us today to see how we can support your development needs!